In this post, I’ll be working in Java to walk through one of the advanced features, Selenium cookies. I’ll start by showing you the Selenium cookies Java class. Next, I’ll explain an example of how you might test a website and see which Java methods you can use. Throughout this post, I’ll be assuming you’re already somewhat familiar with Java, cookies, Selenium, and automated testing concepts.
Where to Find Information About Selenium Cookies
One of the standard features websites use to store user information is cookies. These cookies can contain, for example, information about a visitor’s shopping preferences.
When working with cookies, let’s first open up Chrome’s developer tools. Next, click on the application tab and scroll down to the storage section. Underneath the storage section, you’ll find cookies and their attributes (e.g., Domain, Expires, HttpOnly, etc.). The Value attribute is where the user information will be stored. You can see that the shopping_currency cookie, for example, contains AUD.
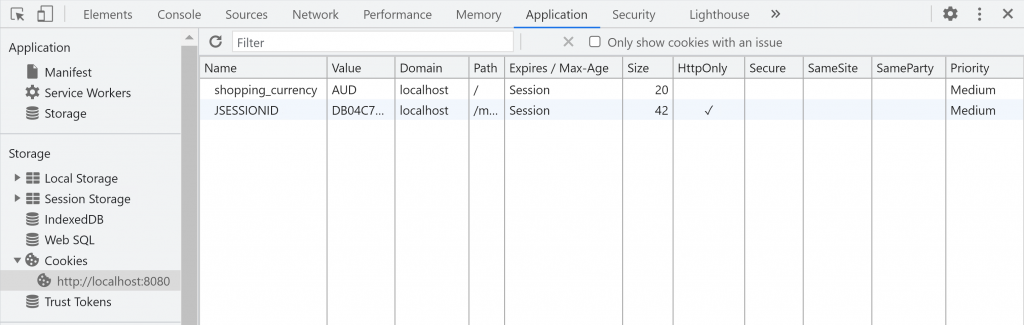
There’s a corresponding getter method for each of the cookie’s attributes in the Selenium cookies class shown in the screenshot below. For example, if you want to check the HttpOnly cookie attribute, you can call the isHttpOnly method. However, there currently aren’t getter methods for the SameSite, SameParty, and Priority cookie attributes. One possible reason is that they’re not supported on all browsers. Nevertheless, for the SameSite field, a new method, getSameSite, is arriving in Selenium v4, which is currently in beta. The screenshot below is from v3.141.59.
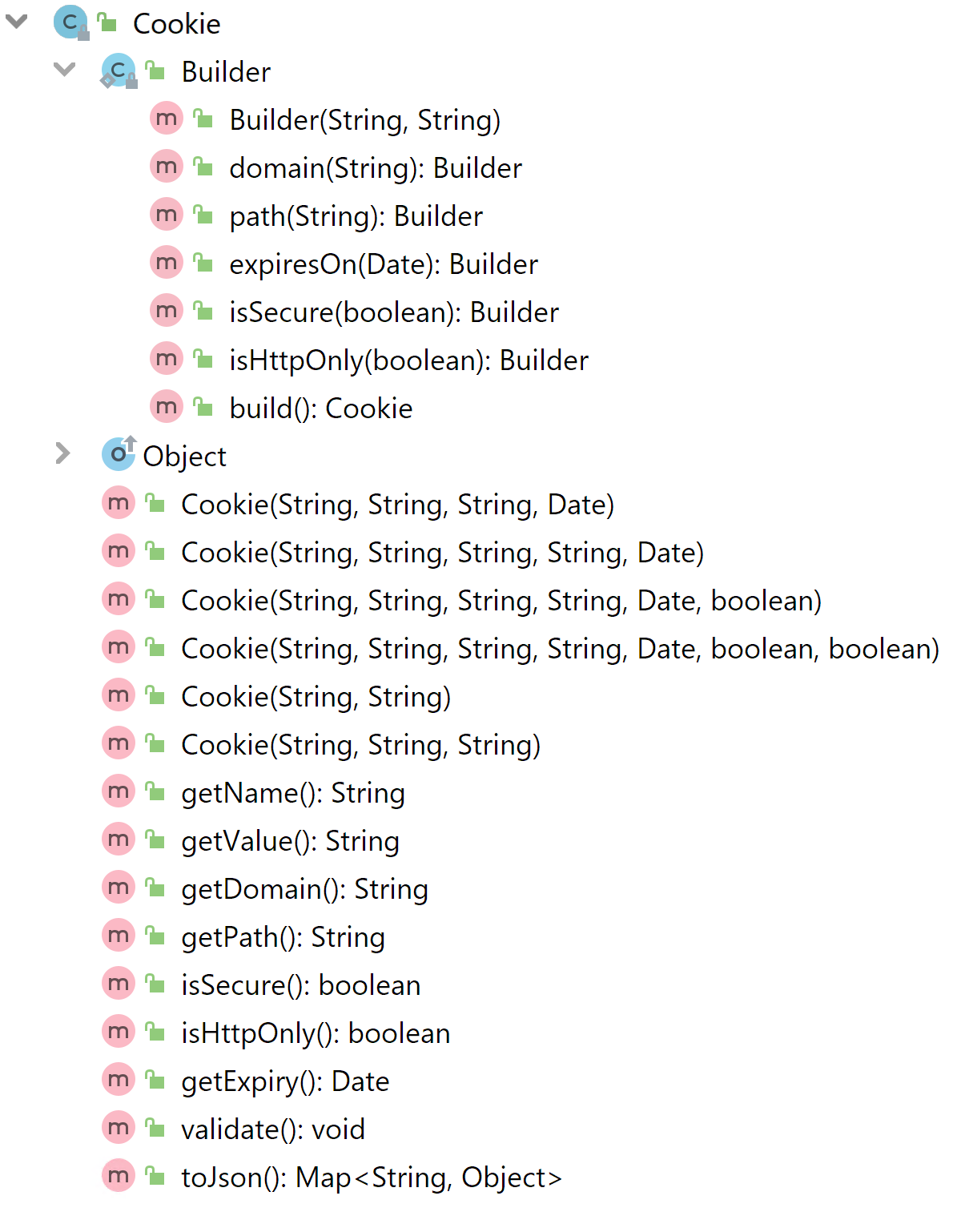
How to Create Selenium Cookies
There are two ways you can create Selenium cookies. The first way is to call the cookie’s constructor, passing in at least a key and value argument (i.e., new Cookie(“KEY”, “VALUE”) ). The second way is to use the inner class Builder to quickly chain methods to create a new cookie object.
Cookie newCookie = new Cookie .Builder(originalCookie.getName(),"NEW COOKIE VALUE") .domain(originalCookie.getDomain()) .expiresOn(originalCookie.getExpiry()) .path(originalCookie.getPath()) .isSecure(originalCookie.isSecure()) .build();
Being able to use Builder to create new cookies will be important later on. For example, we’ll use it to copy information from an existing cookie into a new cookie. That’s because, as you may have noticed, you cannot edit an existing cookie. Therefore, to update a cookie’s information, you’ll always need to create a new Selenium cookie object.
Now that you have a basic understanding of the Selenium cookies class let’s see what methods we can use.
Expand Your Test Coverage
Where to Find Methods to Use Selenium Cookies
Let’s start by looking at the get, save, and delete methods in Selenium WebDriver’s Options interface. To access the Options object, you can call manage on an existing WebDriver object (i.e., webDriver.manage() ). The manage method then returns an object that implements the Options interface. This is so that different browsers, such as Chrome, Firefox, or Internet Explorer, can implement their versions of the WebDriver interface.
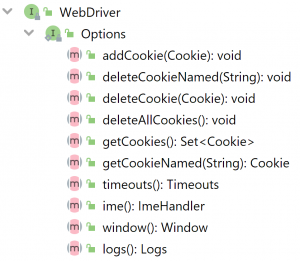
We can see that there are a total of 14 methods in the Options interface. However, we only care about these six methods:
- addCookie
- deleteCookieNamed
- deleteCookie
- deleteAllCookies
- getCookies
- getCookieNamed
To help you understand which methods to use and when to use them, I believe it’s helpful to define an example test scenario.
How to Use Selenium Cookies Methods: An Example
Let’s begin by considering what steps you might take as a developer to test a website’s cookie feature. Afterward, I’ll explain how you’ll automate the testing.
To simplify the Java code in the following sections, I’ve left out information on setting up Maven and importing the Selenium libraries. However, if you need some background on doing this, you can read this blog post on Selenium’s ExpectedConditions. Once you have that set up, you can execute the code in Java’s main method.
As an example, let’s consider the following test scenario. You need to ensure the website remembers a visitor’s shopping preference. This preference will be stored inside a cookie and be used to display prices in a specific currency. The website will also need to handle both new and returning visitors.
You can represent the example test scenario with the following testing steps:
- You add a test cookie, and the website displays the correct currency.
- The website creates a new cookie with currency preferences, and you check if it’s correct.
- You check that the website will use default settings when a cookie is missing.
- After you update a cookie, the website updates correctly.
Now, with the above steps, let’s see some code that can automate the testing for you.
Test Step 1: You Add a Test Cookie, and the Website Displays the Correct Currency
First, you’ll want to make sure that the website can fetch and display the currency preferences from the cookie. Therefore, you’ll need to add a new cookie with some test data. Since we want to automate this, let’s look at how we can do this with the Selenium cookies method addCookie.
WebDriver webDriver = new ChromeDriver(); webDriver.get("http://localhost:8080/ecommerce"); webDriver.manage().addCookie(new Cookie("shopping_currency", "AUD")); webDriver.navigate().refresh(); Assert.assertEquals("Expecting preferences", "AUD", webDriver.findElement(By.xpath("//div[text()='Prices shown in: ']")).getText())
The first line of the code instantiates a new WebDriver object, in this case, a Chrome browser instance. This allows us to fetch the URL page we want to test using the get method.
Next, you can add a Selenium cookie into the browser with the addCookie method, passing in a reference to a newly created cookie. Afterward, when we refresh the webpage with webDriver.navigate.refresh(), we expect the cookie information to be displayed. As we’re using Selenium, you’ll be able to search for the displayed information with XPath. If you need a refresher on XPath, please see this post.
Test Step 2: The Website Creates a New Cookie With Currency Preferences, and You Check If It’s Correct
The next step is to ensure the website correctly saves the user’s currency preferences into a cookie. Therefore, you’ll need to make sure that
- A cookie exists
- It contains the correct information
This time, we’ll use the getCookies and getCookieNamed methods to check whether the shopping_currency cookie exists and contains the correct currency information.
WebDriver webDriver = new ChromeDriver(); webDriver.findElement(By.xpath("//div[text()='Prices shown in: ']")) .findElement(By.xpath("//div[text()='CAD']")).click(); Optional c1 = webDriver.manager().getCookies().stream() .filter(e -> e.getName().equals("shopping_currency")) .findAny(); Assert.assertTrue(c1.isPresent()); Assert.assertEqual("Expecting preferences: ", "CAD", c1.get().getValue());
The first line is the same as before. You’re simulating a user clicking on CAD as the currency to display prices in the second line. On the third line, you can see the getCookies method used to return all the cookies in the browser. This means you’ll need to filter for the cookie you want. We then check that the cookie isPresent and make sure it has the correct information with getValue.
WebDriver webDriver = new ChromeDriver(); webDriver.findElement(By.xpath("//div[text()='Prices shown in: ']")) .findElement(By.xpath("//div[text()='CAD']")).click(); Cookie c2 = webDriver.manage().getCookieNamed("shopping_currency"); Assert.assertNotNull(c2); Assert.assertEqual("Expecting preferences: ", "CAD", c2.getValue());
Instead of having to filter for the specific cookie you want, the other method, getCookieNamed, is the easier way to get a cookie from the browser. You just need to give it a String argument for the name of the cookie you want to fetch. It then returns null if the cookie doesn’t exist. Because we want the cookie to exist, we can assertNotNull.
Test Step 3: You Check That the Website Will Use Default Settings When a Cookie Is Missing
Now, in this step, we’ll need to make sure of two things. First, we need to make sure the website doesn’t crash when there’s no cookie. Second, the website must default back to using USD when the cookie is missing. To make sure of that, you’ll need to delete cookies from the browser. This time, we have the deleteCookie, deleteCookieNamed, and deleteAllCookies methods.
WebDriver webDriver = new ChromeDriver(); Cookie c3 = webDriver.manage().getCookieNamed("shopping_currency"); webDriver.manage().deleteCookie(c3); webDriver.manage().deleteCookieNamed("shopping_currency"); webDriver.manage().deleteAllCookies(); webDriver.navigate().refresh(); Assert.assertEquals("Expecting default currency", "USD", webDriver.findElement(By.xpath("//div[@text="Prices shown in: ")).getText())
Similar to fetching cookies, the second method, deleteCookieNamed, is the easiest method to use. Again, you just need to provide the method with the cookie’s name you want to delete. The first method, deleteCookie, is a little trickier because you’ll need to give it a reference to an existing cookie object. That’s why we call getCookieNamed in the line before. Nevertheless, if you want to delete all cookies in the browser, you can call deleteAllCookies.
After you’ve deleted the cookies, you’ll need to check if it’s behaving correctly. The method findElement will throw an ElementNotFoundException if the website crashes or if the element is not rendered, while assertEquals will fail if the currency information is incorrect.
Test Step 4: After You Update a Cookie, the Website Updates Correctly
As I mentioned in the section on how to create Selenium cookies, you cannot edit cookies. Therefore, to update the value inside a cookie, you’ll need to delete and re-add them. Because I’ve already covered how to delete and add Selenium cookies, I’ll show you how to use them together. If you delete and add a cookie with the same name, the effect will be the same as editing a cookie.
WebDriver webDriver = new ChromeDriver(); Cookie originalCookie = webDriver.manage().getCookieNamed("shopping_currency"); webDriver.manage().deleteCookie(originalCookie); Cookie newCookie = new Cookie .Builder(originalCookie.getName(),"NEW COOKIE VALUE") .isSecure(originalCookie.isSecure()) .path(originalCookie.getPath()) .build(); webDriver.manage().addCookie(newCookie); Assert.assertEquals("Expecting preferences", "NEW COOKIE VALUE", webDriver.findElement(By.xpath("//div[@text="Prices shown in: ")).getText())
First, you’ll need to grab the original cookie you want to edit with getCookieNamed. Next, you delete the cookie with the deleteCookie method. Afterward, you create a new cookie with Builder and copy across the information you need. Finally, you’ll add the cookie back with addCookie. We then assertEquals to ensure the website has updated itself with the information in the updated cookie.
Conclusion
This post has shown you how to use Selenium cookies methods to test an example cookie feature on a website. I also gave a brief description of the Selenium cookies class and methods to get cookie information.
Now, if all this automated testing seems a little too complicated and you want to just focus on development, don’t forget to check out Testim for a free account. Testim can help you easily take care of testing cookies, as it offers custom steps for clearing cookies and many other features. So, now there are no more excuses for your website to have any broken cookie features.
What to read next
How Do I Test iFrames With Selenium?
Selecting an Element in Selenium: A Beginner’s Walk-Through