Code coverage guides us toward thoroughly tested and, ultimately, high quality code. And it’s with code coverage tooling that we’re able to understand the status of our current coverage.
If you’re currently using Mocha to test your application, you’re in luck. Today we’re going to cover everything you need to know about getting set up with Mocha code coverage using a tool called Istanbul.
By the end of this post, you’ll know how to configure code coverage in Mocha, how coverage works, how to use code coverage metrics, and some techniques that you can use to make the most of your coverage.
Sound good? Let’s get to it.
How We’ll Break Down Code Coverage
Before we jump into the details, let’s briefly go over how we’ll break down today’s post.
- Get started with Istanbul and Mocha. Our start guide will get you set up quickly and easily if you’re in a rush.
- What is Istanbul and how does it work? We’ll cover how Istanbul works under the hood.
- How to take Istanbul further. We’ll look at the more advanced features and uses of code coverage.
Now, let’s jump straight in with the quick start.
Mocha With Istanbul: A Quick Start
Assuming you already have Mocha on your machine, setting up Mocha and Istanbul takes only two steps.
Step 1: Install NYC
NYC is Istanbul’s command line utility. The first thing you’ll need to do is install NYC:
npm install nyc --save-dev
Step 2: Use NYC to Call Mocha
With Istanbul installed, prepend your existing Mocha command with the NYC binary. For instance, your package.json would look like the following:
{ "scripts": { "test": "nyc mocha" } }
Now when you run your test command, you’ll see the following output of ASCII coverage data following your test report:
(If you’re confused about the different metrics, don’t worry. We’ll cover those later in the post, so read on.)
And that’s all there is to it.
But since that was very quick, let’s break down the NYC command and what it’s doing to have a look at what’s going on here.
What Is Istanbul and How Does It Work?
Let’s start by looking at what Istanbul is.
What Is Istanbul?
Istanbul is a test coverage tool that works with many different frameworks. It tracks which parts of your code are executed by your unit tests. Thus, you can use Istanbul to view and see coverage gaps, or you can integrate it integrated into your CI pipeline to enforce coverage levels. Ultimately, Istanbul enables data-driven testing.
The NYC package is Istanbul’s CLI tool that makes integrating with Istanbul easier, no matter your current tooling. You might be wondering why it has such an obscure name. And the answer is because of some totally bizarre reasoning related to a song about Istanbul. So don’t worry—it’s not just you that was confused.
And lastly, before we look at Istanbul in more detail, it’s worth remembering that Istanbul is generic. Istanbul works with many different unit test libraries, including Mocha. So there aren’t any nuances that you’ll need to know about Mocha and Istanbul together beyond just getting NYC set up.
How Does Istanbul Coverage Work?
Istanbul isn’t voodoo. But understanding how it works will help us harness the tool to greater effect.
Istanbul collects coverage by wrapping various functions inside the JavaScript language so that when your code is invoked, so too is Istanbul’s monitoring code. By wrapping code the way Istanbul does, we are able to see coverage on a granular level, like which branches have been invoked.
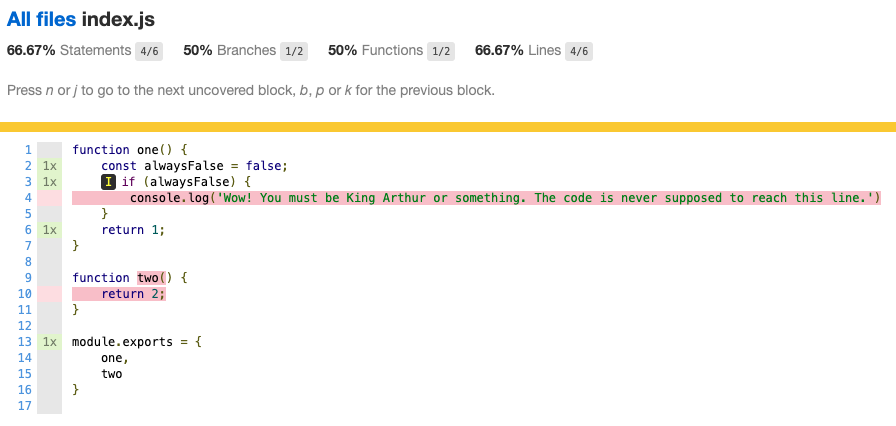
Now, if you’re wondering where we got this magical view of our coverage, it’s called a coverage reporter. We’ll discuss that shortly. But first, let’s take a look at what those different metrics mean.
Making Sense of the Istanbul Output
One other thing you might have noticed is that Istanbul’s output has more than one metric. It’s not a simple coverage value. So what do these different metrics mean?
- Statements: The individual “blocks of code” that are covered.
- Branch: The conditionals “forks” (if/else) in the code that are covered.
- Functions: The percentage of functions invoked in the tests.
- Lines: The amount of actual physical lines that are covered by tests.
The metric you choose to monitor is up to you. You can use all the metrics together if you want a more well-rounded picture of your current coverage.
Taking Istanbul Further
By now, you should have a good handle on how to set up Istanbul, and you should have a good idea about how it works. Now we’re going to discuss a few advanced ways that we can take our Istanbul tooling further.
Setting Up an HTML Reporter
The main way to get additional value from your Istanbul setup is to use it with a test reporter.
A reporter is simply a different way of viewing your test output other than the ASCII dump that we saw before. One of the most popular reporters is an HTML reporter. HTML reporters are simple to view and can be easily hosted.
Adding an HTML reporter to Istanbul is easy. To get set up with an HTML reporter, all you have to do is add the reporter flag to your NYC command as follows:
nyc --reporter=html mocha
Now when you run your Mocha command, you’re going to see a new coverage folder appear in the root of your project. Inside that folder is an HTML file that shows the latest test run.
At this point, you can either review the coverage file directly in your browser, or you can push the coverage files to a remote server so you can see your coverage more easily and track changes over time.
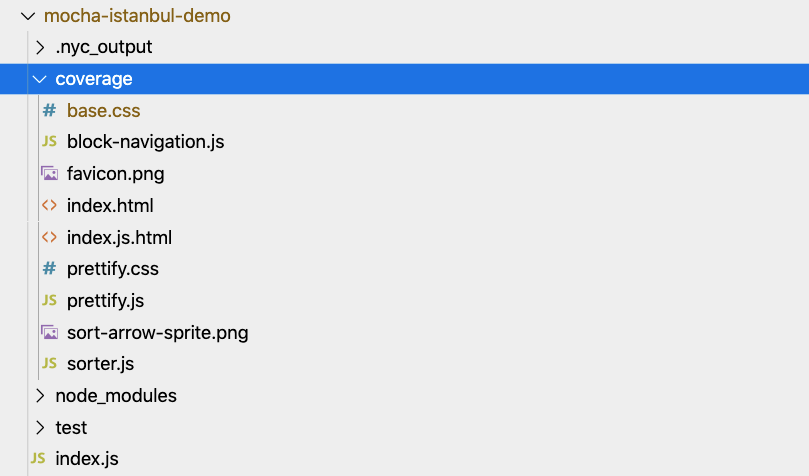
But it’s not just the top level page. If you navigate to the coverage directory we discussed, you can now open the index.html that was generated to see the full report.
Then, if you click on the files, you should see a breakdown of the individual file itself. So you can see the different parts of the application that aren’t tested fully and could need some attention.
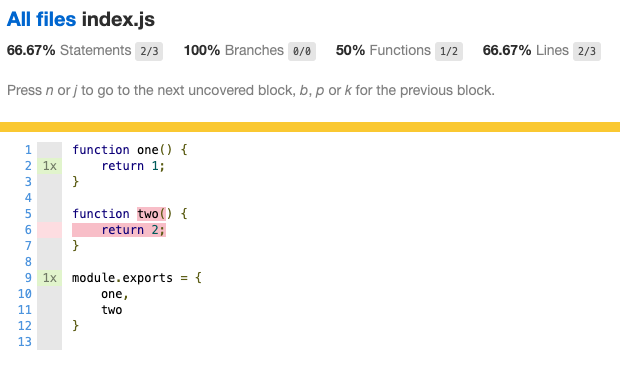
Integrating Coverage Into CI
Another great way to get the most out of Istanbul coverage is to implement code coverage thresholds. A threshold sets a percentage value that your coverage must be higher than. If coverage drops below the predefined level, then an error is thrown.
Thresholds are useful to ensure that all checked in code passes the standard that your team set for your codebase. In order to get set up with coverage thresholds, add the following to your package.json:
{ "nyc": { "branches": ">80", "lines": ">80", "functions": ">80", "statements": ">80" } }
Next, update your Istanbul command to pass the flag:
--check-coverage=true
Now you should be able to define the thresholds that you want for your application and programmatically enforce your standards.
Good Luck Raising Your Code Coverage
And that concludes today’s walkthrough of setting up Istanbul with Mocha.
As you raise your coverage levels, you will see a drastic impact on the quality of your codebase. And having metrics on hand really makes it much easier to manage and stay on top of healthy coverage levels.
I hope this post helped cleared up the uncertainty about how you can get Mocha code coverage set up using Istanbul. Not only are you now set up with coverage tooling, but you should also have a good idea about how to leverage other features of Istanbul, such as test reporting and CI threshold integrations, in order to move your coverage efforts forward.
Good luck testing your application!
This post was written by Lou Bichard. Lou is a JavaScript full stack engineer with a passion for culture, approach, and delivery. He believes the best products emerge from high performing teams and practices. Lou is a fan and advocate of old-school lean and systems thinking, XP, continuous delivery, and DevOps.